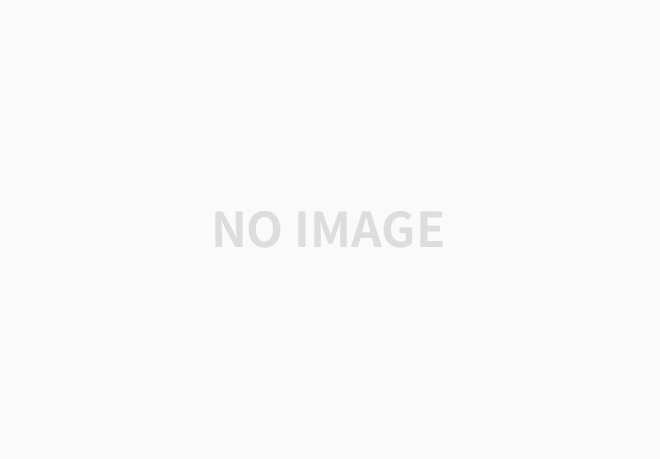
0. C# _ 상속 및 접근제어에 관해서
- 상속 : 부모 클래스의 내용을 상속받은 자식 클래스가 물려받는것. 재사용 및 확장에 용이
* 자식 클래스에서 부모 클래스가 가지고 있는 내용을 사용 가능
- 접근 제어 : 부모 클래스의 내용을 자식 클래스 및 외부에서 접근하는 권한을 제어
[ 이 예시의, 상속과 접근 제어 구조 ]
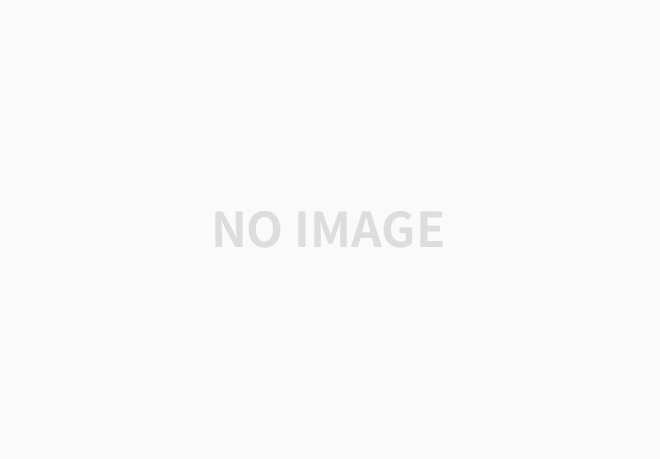
1. UI 부분
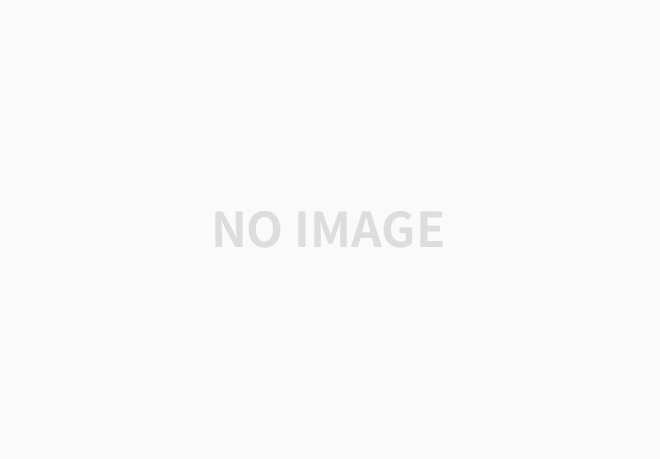
2. 코드 부분
2-1 ) CBase 클래스
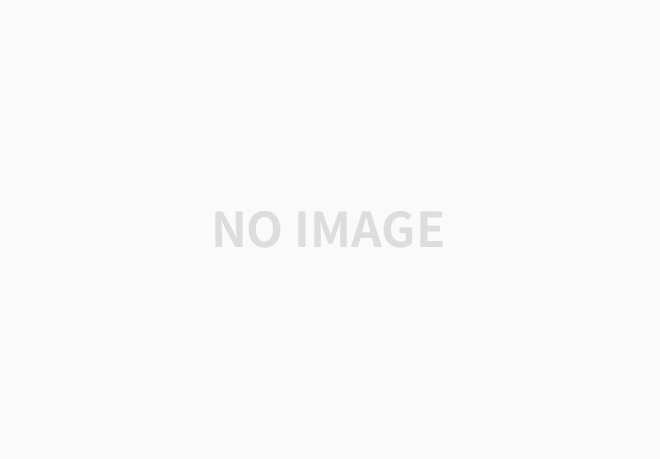
using System;
using System.Collections.Generic;
using System.Drawing; // Pen을 쓰기 위해서는, 이것이 있어야 함.
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace _20210608_inheritance // 네임스페이스 일치하는지 확인하기
{
class CBase
{
public string strName; // 이름 변수
protected Pen _Pen; // Pen 클래스의, _Pen 객체
// private : 이 클래스 내부에서만, Pen을 쓸 수 있음. 상속받은 애들 또한 볼 수 없음.
// Protected : 외부에서 볼수없음. 그러나, 상속받은 애들은 볼 수 있음.
public CBase() // 생성자
{
_Pen = new Pen(Color.Aqua);
}
}
}
2-2 ) COneCycles 클래스
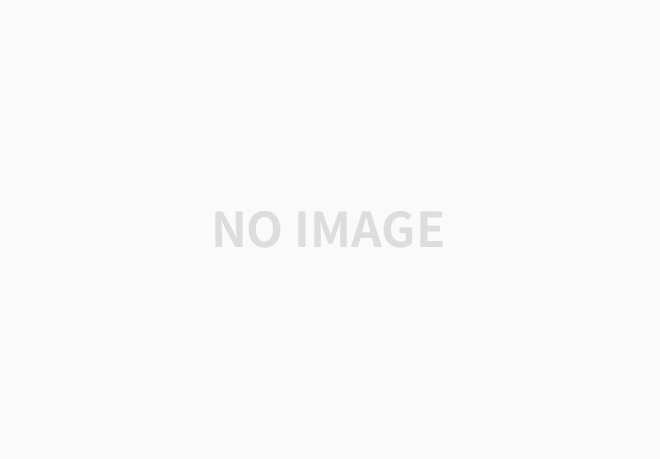
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Drawing;
namespace _20210608_inheritance
{
class COneCycles : CBase // COneCycles는 CBase를 상속받아 만든 클래스이다.
{
public Rectangle _rtCircle1; // 바퀴 1
public Rectangle _rtSquare1; // 몸통 1
public COneCycles(string sName) // 생성자
{
strName = sName; // strName은 CBase에 존재하는 변수이므로, 상속받아 사용 가능
_Pen = new Pen(Color.WhiteSmoke,3); // Pen(색상, 너비, 굵기)
_rtCircle1 = new Rectangle(120, 150, 120, 120);
_rtSquare1 = new Rectangle(150, 30, 60, 120);
}
public Pen fPenInfo() // 해당 객체에 대한, 펜 정보 반환 메소드
{
return _Pen;
}
[ 외부에서 호출 가능하도록 ]
public void fMove(int iMove) // 펜 이동 메소드 (바퀴 이동 메소드 + 몸통 이동 메소드)
{
fCircle1Move(iMove);
fSquare1Move(iMove);
}
[ 내부에서만 움직인다 ]
protected void fCircle1Move(int iMove) // 바퀴 이동 메소드
{
Point oPoint = _rtCircle1.Location;
oPoint.X = oPoint.X + iMove; // iMove만큼 움직인다
_rtCircle1.Location = oPoint;
}
protected void fSquare1Move(int iMove) // 몸통 이동 메소드
{
Point oPoint = _rtSquare1.Location;
oPoint.X = oPoint.X + iMove;
_rtSquare1.Location = oPoint;
}
}
}
2-3 ) CCycle 클래스
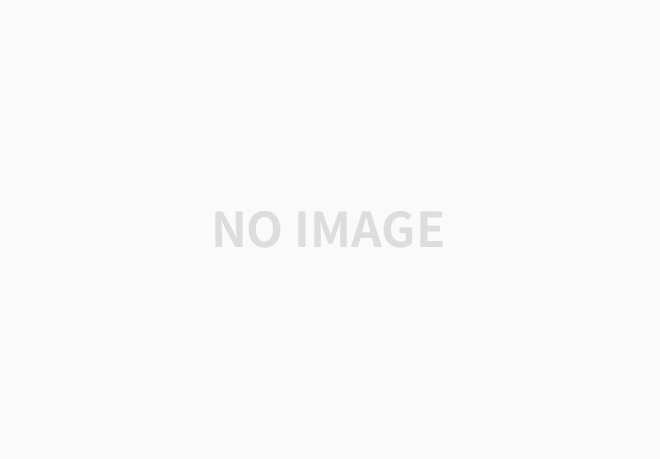
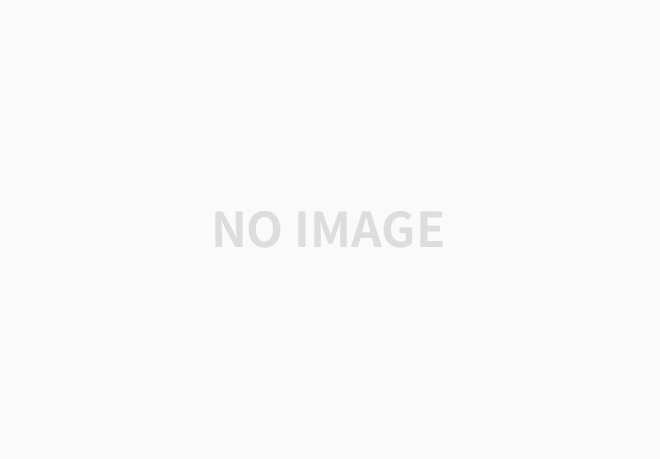
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Drawing;
namespace _20210608_inheritance
{
class CCycle : CBase
{
public Rectangle _rtCircle1; // 바퀴 1
public Rectangle _rtCircle2; // 바퀴 2
public Rectangle _rtSquare1; // 몸통 1
public CCycle(string sName) // 생성자
{
strName = sName; // 이름을정의할필요가없음 상속받아서tring strName의값이옴
_Pen = new Pen(Color.Black, 3); // 색상 너비굵기
_rtCircle1 = new Rectangle(30,150,120,120);
_rtCircle2 = new Rectangle(210,150,120,120);
_rtSquare1 = new Rectangle(60,90,240,60);
}
public Pen fPenInfo() // 해당 객체에 대한, 펜정보 반환 메소드
{
return _Pen;
}
[ 외부에서 호출 가능하도록 ]
public void fMove(int iMove) // 펜 이동 메소드 (바퀴 1/2 이동 메소드 + 몸통 이동 메소드)
{
fCircle1Move(iMove);
fCircle2Move(iMove);
fSquare1Move(iMove);
}
[ 내부에서만 움직인다 ]
protected void fCircle1Move(int iMove) // 바퀴 1 이동 메소드
{
Point oPoint = _rtCircle1.Location;
oPoint.X = oPoint.X + iMove;
_rtCircle1.Location = oPoint;
}
protected void fCircle2Move(int iMove) // 바퀴 2 이동 메소드
{
Point oPoint = _rtCircle2.Location;
oPoint.X = oPoint.X + iMove;
_rtCircle2.Location = oPoint;
}
protected void fSquare1Move(int iMove) // 몸통 1 이동 메소드
{
Point oPoint = _rtSquare1.Location;
oPoint.X = oPoint.X + iMove;
_rtSquare1.Location = oPoint;
}
}
}
2-4 ) CCar 클래스
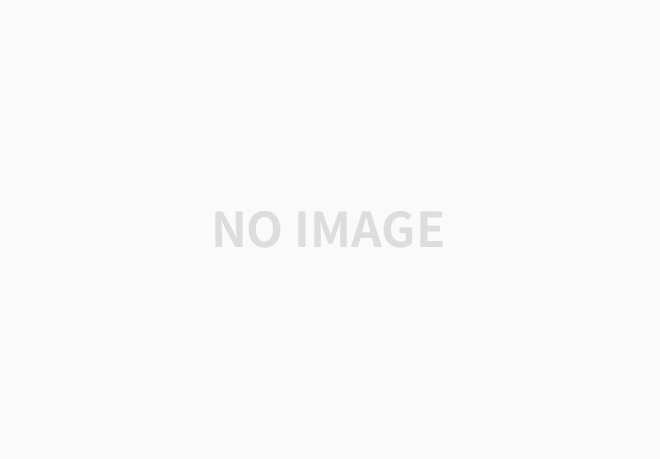
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Drawing;
namespace _20210608_inheritance
{
class CCar : CCycle // CCycle을 상속받음
{
public Rectangle _rtSquare2; // 몸통
public CCar(string sName) :base(sName) // 생성자
{
strName = sName;
_Pen = new Pen(Color.Blue, 3); // 색상 너비굵기
_rtCircle1 = new Rectangle(60, 180, 90, 90);
_rtCircle2 = new Rectangle(210, 180, 90, 90);
_rtSquare1 = new Rectangle(90,30,180,90);
_rtSquare2 = new Rectangle(30, 120, 300, 60);
}
[ 외부에서 호출 가능하도록 ]
public new void fMove(int iMove) // 상속받은 이름이랑 같기 때문에 new를쓰자
{
fCircle1Move(iMove); // 상속받음
fCircle2Move(iMove); // 상속받음
fSquare1Move(iMove); // 상속받음
fSquare2Move(iMove);
}
protected void fSquare2Move(int iMove) // 몸통 2 이동 메소드
{
Point oPoint = _rtSquare2.Location;
oPoint.X = oPoint.X + iMove;
_rtSquare2.Location = oPoint;
}
}
}
2-5 ) Form
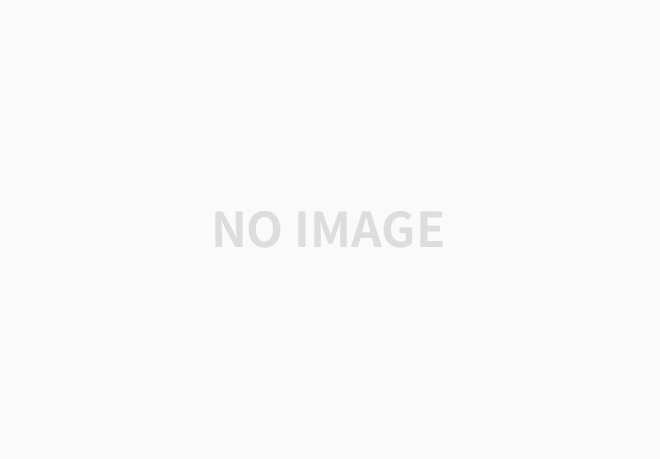
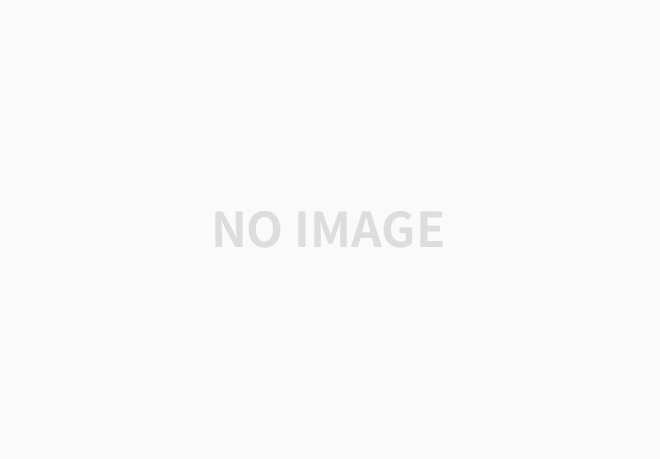
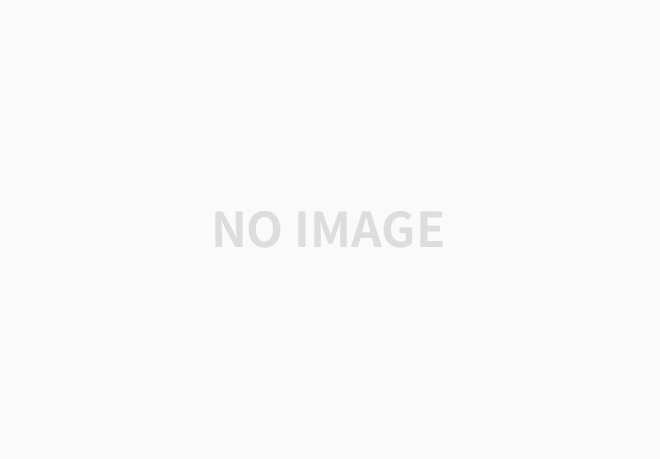
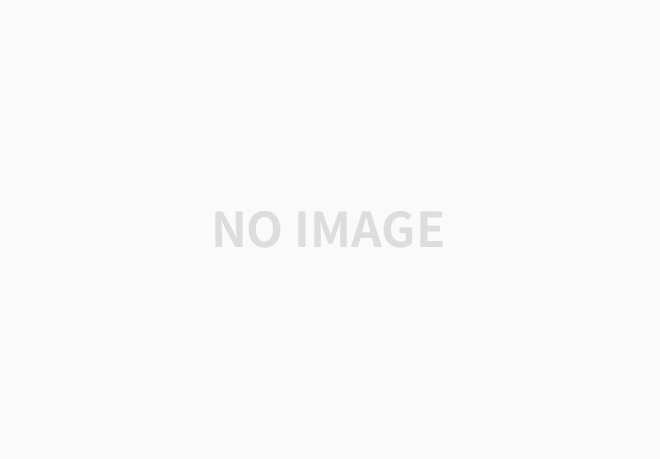
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace _20210608_inheritance
{
public partial class Form1 : Form
{
COneCycles _c0C; // 전역변수로 객체 생성
CCycle _cC;
CCar _cCar;
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) // 폼을 로드할 때
{
_c0C = new COneCycles("외발자전거 "); // 이름을 넣어줌으로써, 코드로 작성해준 객체 생성자 실행
_cC = new CCycle("자전거 ");
_cCar = new CCar("자동차 ");
}
private void btnOneCycle_Click(object sender, EventArgs e) // OneCycles버튼을 클릭했을 때
{
fClearPanel();
OneCycleDraw();
}
private void OneCycleDraw() // OneCycle에대한 위치 그림을 그려준다
{
lblName.Text = _c0C.strName; // _c0C의 이름을, lblName의 Text부분에 표시한다.
Graphics g = PMain.CreateGraphics(); // Panel(PMain)에 그림을 그릴 것이며, 이 정보를 g객체에 저장한다.
Pen p = _c0C.fPenInfo(); // _c0C 객체의 펜에대한 정보를 가져와서, p객체에 저장해준다.
g.DrawRectangle(p, _c0C._rtSquare1); // 사각형 그리기 (p펜으로, _c0C객체에 저장된 값으로)
g.DrawEllipse(p, _c0C._rtCircle1);
}
private void btnCycle_Click(object sender, EventArgs e) // Cycle 버튼을 클릭했을 때
{
fClearPanel();
CCycleDraw();
}
private void CCycleDraw() // Cycle에 대한 위치 그림을 그려준다.
{
lblName.Text = _cC.strName;
Graphics g = PMain.CreateGraphics();
Pen p = _cC.fPenInfo();
g.DrawRectangle(p, _cC._rtSquare1);
g.DrawEllipse(p, _cC._rtCircle1);
g.DrawEllipse(p, _cC._rtCircle2);
}
private void btnCar_Click(object sender, EventArgs e) // Car버튼을 클릭했을 때
{
fClearPanel();
CarDraw();
}
private void CarDraw() // OneCycle에대한위치그림을그려준다
{
lblName.Text = _cCar.strName;
Graphics g = PMain.CreateGraphics();
Pen p = _cCar.fPenInfo();
g.DrawRectangle(p, _cCar._rtSquare1);
g.DrawRectangle(p, _cCar._rtSquare2);
g.DrawEllipse(p, _cCar._rtCircle1);
g.DrawEllipse(p, _cCar._rtCircle2);
}
private void fClearPanel() // 판넬 새로 그려주기
{
PMain.Invalidate(); // 컨트롤의 전체 화면을 무효화하고, 컨트롤을 다시 그린다.
Refresh(); // 컨트롤이 해당 컨트롤의 클라이언트 영역을 강제로 무효화하도록 하고, 컨트롤 자체와 모든 자식 컨트롤을 즉시 다시 그리도록 한다.
}
private void btnLeft_Click(object sender, EventArgs e) // Left 버튼을 눌렀을 때
{
fClearPanel();
switch (lblName.Text)
{
case "외발자전거 ":
_c0C.fMove(-5);
OneCycleDraw();
break;
case "자전거 ":
_cC.fMove(-5);
CCycleDraw();
break;
case "자동차 ":
_cCar.fMove(-5);
CarDraw();
break;
default:
break;
}
}
private void btnRight_Click(object sender, EventArgs e) // Right 버튼을 눌렀을 때
{
fClearPanel();
switch (lblName.Text)
{
case "외발자전거 ":
_c0C.fMove(5);
OneCycleDraw();
break;
case "자전거 ":
_cC.fMove(5);
CCycleDraw();
break;
case "자동차 ":
_cCar.fMove(5);
CarDraw();
break;
default:
break;
}
}
}
}
3. 결과물 부분

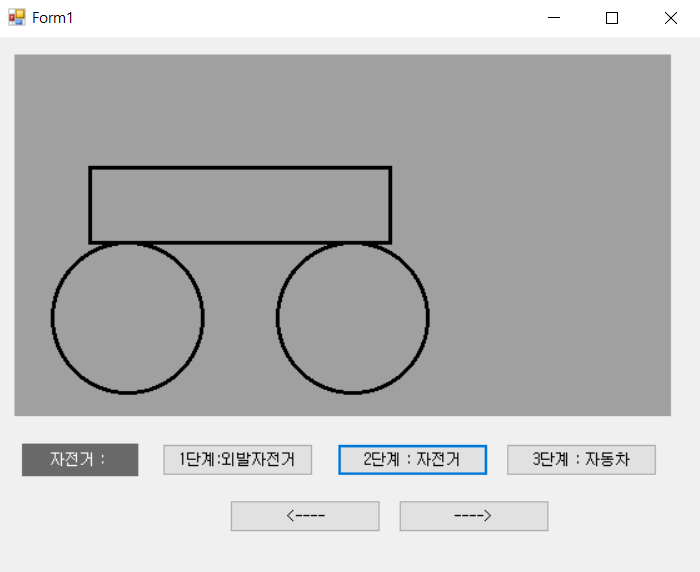
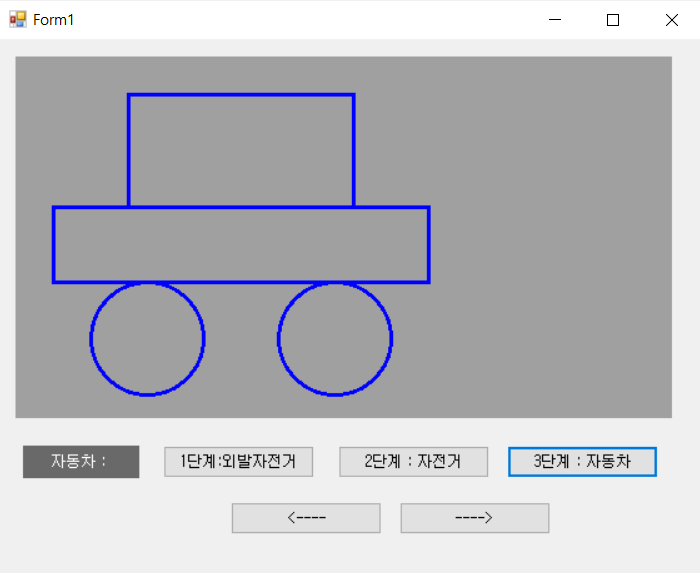


4. C#기초 예시 / 참고 자료를 더 보고 싶다면?
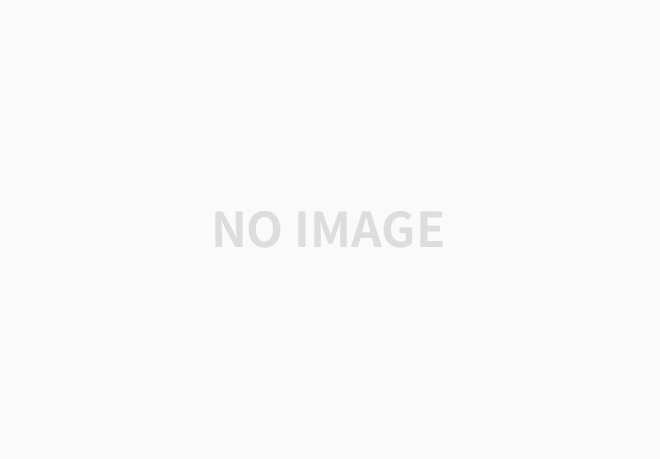
* 유의사항 - 아직 공부하고 있는 문과생 코린이가, 정리해서 남겨놓은 정리 및 필기노트입니다. - 정확하지 않거나, 틀린 점이 있을 수 있으니, 유의해서 봐주시면 감사하겠습니다. - 혹시 잘못된 점을 발견하셨다면, 댓글로 친절하게 남겨주시면 감사하겠습니다 :) |
'문과 코린이의, [C#] 기록 > C# 활용' 카테고리의 다른 글
[문과 코린이의 IT 기록장] C# 기초 예시 - StreamReader, StreamWrite (0) | 2021.06.26 |
---|---|
[문과 코린이의 IT 기록장] C# 기초 예시 - 오버라이딩과 오버로딩 (0) | 2021.06.25 |
[문과 코린이의 IT 기록장] C# 기초 예시 - 클래스(Class) : 생성자, 소멸자 (1) | 2021.06.10 |
[문과 코린이의 IT 기록장] C# 기초 예시 - 구조체(Struct), 클래스(Class) (1) | 2021.06.09 |
[문과 코린이의 IT 기록장] C# 기초 예시 - 반복문2 (While문, DoWhile문) 활용 (0) | 2021.06.08 |